Table Of Content
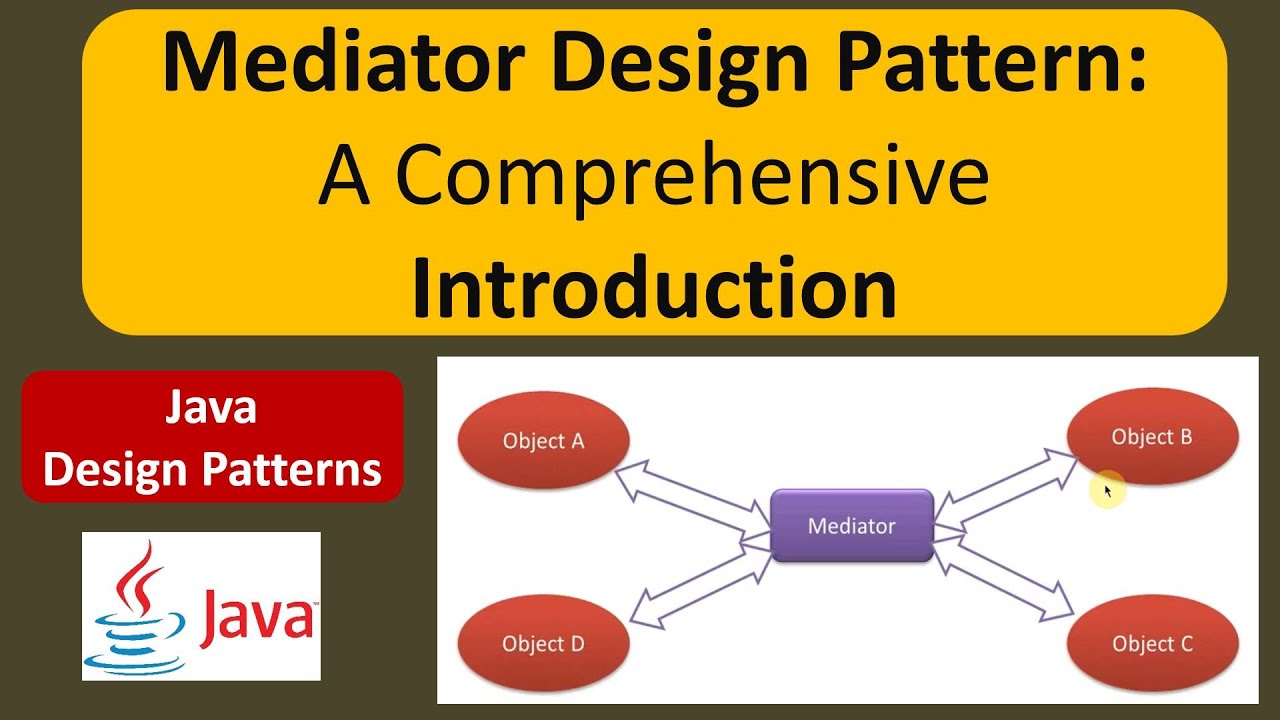
In Mediator, a mediator adds functionality and thus is known by the colleague objects. Facade, on the other hand, defines a simplified interface to a subsystem; it does not introduce additional functionality and is unknown to the subsystem classes. According to the Gang of Four definitions, define an object that encapsulates how a set of objects interact with each other. Mediator promotes loose coupling by keeping objects from explicitly referring to each other and letting you vary their interaction independently.
DESIGN PATTERNS SAGA #13: REAL PROJECT SITUATIONS WITH MEMENTO
Student talks to Teacher, Teacher manages the communication, and the information goes back through the Teacher to the requesting student. This way, everyone collaborates effectively, and individual students don’t have to directly deal with all the details of each other’s work. With our example, let’s look at the participants of the Mediator pattern. The primary difference is in the type of interaction between modules. I prefer to keep the code part separated, so, I have already updated this GitHub repository with the implementation of the Mediator Pattern from scratch.
Participants of the Mediator Pattern
They communicate through the Mediator, and each colleague class is only aware of the mediator, not the other colleagues. This isolation ensures that changes in one colleague do not directly affect others. It defines how a mediator object facilitates the interaction between other objects, guiding their behavior and communication without them being directly aware of each other. This pattern emphasizes the behavior and communication patterns among objects. It is the mediator object that encapsulates all interaction logic between objects in a system. Therefore, if an existing object is updated with new interaction rules or a new object is added to the system, it is only the mediator object that you need to update.
Design Patterns
Say you have a dialog for creating and editing customer profiles. It consists of various form controls such as text fields, checkboxes, buttons, etc. However, you’ll likely have to support other means of transport as well.
Mediator Pattern Example in JDK
As shown in the image below, here we introduce the Mediator object. The mediator object here will receive the message from each object and route that message to the destination object. So, the mediator object will take care of handling the messages.
When I'm building a GUI I don't like each control knowing each other because that would require subclassing. In Spring MVC, there is a great example of the Mediator Pattern in action with how Spring MVC uses the Dispatcher Servlet in conjunction with the controllers. Imagine a war zone where armed units are moving into the enemy’s territory. Armed units can include soldier, tank, grenadier, and sniper units.
UML Class Diagram
Thus, instead of being tied to a dozen form elements, the button is only dependent on the dialog class. In the Commander interface (Mediator) above, we declared five methods that we implemented in the CommanderImpl class (ConcreteMediator). In the CommanderImpl class, we added two reference to ArmedUnit (Colleague) and initialized them through the registerArmedUnits() method from Line 12 – Line 16.
X-ray light reveals how virus responsible for COVID-19 covers its tracks, eluding the immune system - EurekAlert
X-ray light reveals how virus responsible for COVID-19 covers its tracks, eluding the immune system.
Posted: Tue, 10 Jan 2023 08:00:00 GMT [source]
Mediator Pattern
The Mediator pattern allows us to centralize coordination activities that do not belong in the individual modules. Unlike the Facade pattern, Colleagues are aware of the existence of the Mediator and communicate with the mediator instead of directly with each other. The Mediator itself communicates with Colleagues to fulfill requests. Note that this interface contains the declaration of two methods, AddParticipant and BroadcastMessage. This real-world code demonstrates the Mediator pattern facilitating loosely coupled communication between different Participants registering with a Chatroom.
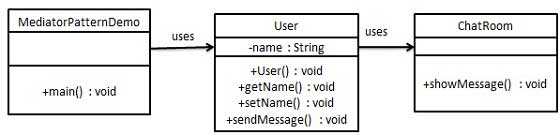
The Mediator pattern promotes a "many-to-many relationship network" to"full object status". Modelling the inter-relationships with an objectenhances encapsulation, and allows the behavior of thoseinter-relationships to be modified or extended through subclassing. The middleware pattern makes it easy for us to simplify many-to-many relationships between objects, by letting all communication flow through one central point. Let us first implement the above Facebook Example using the Mediator Design Pattern in C# step by step.
Instead of letting every objects talk directly to the other objects, resulting in a many-to-many relationship, the object’s requests get handled by the mediator. The mediator processes this request, and sends it forward to where it needs to be. The Mediator makes it easy to modify, extend and reuse individual components because they’re no longer dependent on the dozens of other classes. Components must not be aware of the existence of other components. If anything significant occurs inside or to a component, the component must only alert the mediator. When the mediator receives the notice, it may simply identify the sender, which may be sufficient to determine which component should be invoked in response.
That’s all for mediator design pattern and it’s implementation in java. Mediator design pattern is one of the behavioral design pattern, so it deals with the behaviors of objects. Mediator design pattern is used to provide a centralized communication medium between different objects in a system. This will be an Interface that defines operations that colleague objects can call for communication. So, Create an interface named FacebookGroupMediator.cs and copy and paste the following code. This interface declares two abstract methods (i.e., SendMessage and RegisterUser).

The message sent by any user should be received by all the other users in the group. The mediator design pattern defines an object that encapsulates how a set of objects interact. The Mediator is a behavioral pattern (like the Observer or the Visitor pattern) because it can change the program’s running behavior.
Then, we called called the startAttack() method passing the SoldierUnit and TankUnit objects one after the other. It is only when the test method calls the ceaseAttck() method on Commander passing the currently attacking SoldierUnit object, the TankUnit should be able to attack. We verified this by calling the startAttack() method of Commander passing the TankUnit object. Going ahead with our example on armed units, let’s apply the Mediator pattern to it.